js策略模式:
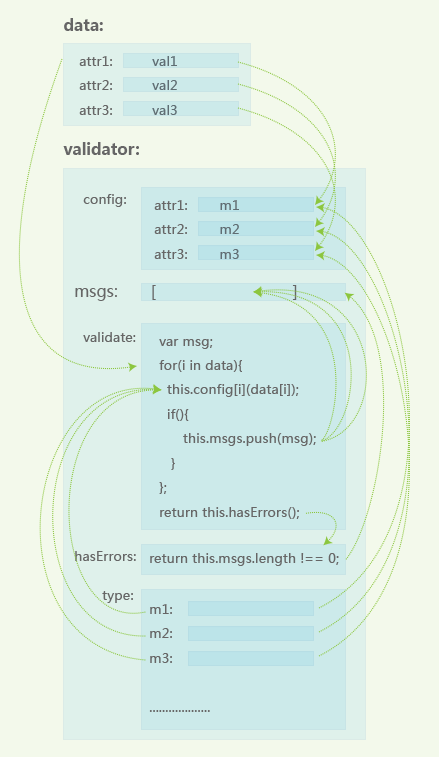
var validator = {
types: {
isNonEmpty: {
validate: function (value) {
return value !== '';
},
instructions: '不能为空'
},
isNumber: {
validate: function (value) {
return !isNaN(value);
},
instructions: '只能为数字'
},
isAlphaNum: {
validate: function (value) {
return !/[^a-z0-9]/i.test(value);
},
instructions: '只能为数字与字母的集合,不能包含特殊字符'
}
},
messages:[],
validate:function(data){
var i,msg,type,checker,result_ok;
this.messages = [];
for(i in data){
if(data.hasOwnProperty(i)){
type = this.config[i];
checker = this.types[type];
if(!type){
continue;
}
if(!checker){
throw {
name:'ValideationError',
message : '缺少验证方法:' + type
}
}
result_ok = checker.validate(data[i]);
if(!result_ok){
msg = '数据'+i+'验证不通过,原因为:'+checker.instructions;
this.messages.push(msg);
}
}
}
return this.hasErrors();
},
hasErrors:function(){
return this.messages.length !== 0;
}
};
var data = {
first_name: 'Super',
last_name: "Man",
age: "unknown",
username: "o_O"
};
validator.config = {
first_name: 'isNonEmpty',
age: 'isNumber',
username: 'isAlphaNum'
};
validator.validate(data);