js代理模式:
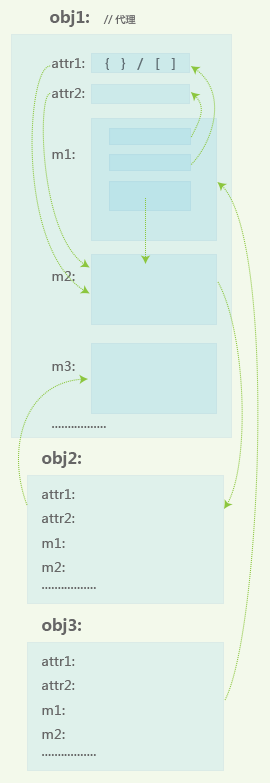
var proxy = {
ids:[],
delay:50,
timeout:null,
callback:null,
context:null,
makeRequest:function(id,callback,context){
this.ids.push(id);
this.callback = callback;
this.context = context;
if(!this.timeout){
this.timeout = setTimeout(function(){
proxy.flush();
},this.delay);
}
},
flush:function(){
http.makeRequest(this.ids,'proxy.handler');
this.timeout = null;
this.ids = [];
},
handler:function(data){
var i,max;
if(parseInt(data.query.count,10)===1){
proxy.callback.call(proxy.context,data.query.results.Video);
return;
}
for(i=0,max=data.query.results.Video.length;i<max;i+=1){
proxy.callback.call(proxy.context,data.query.results.Video[i]);
}
}
};
var http = {
makeRequest:function(ids,callback){
var url = 'http://query.yahooapis.com/v1/public/yql?q=',
sql = 'select * from music.video.id where ids IN ("%ID%")',
format = 'format=json',
handler = 'callback=' + callback,
script = document.createElement("script");
sql = sql.replace('%ID%',ids.join('","'));
sql = encodeURIComponent(sql);
url += sql + '&' + format + '&' + handler;
script.src = url;
document.body.appendChild(script);
}
};
var videos = {
getPlays :function(id){},
updataList:function(data){},
getInfo:function(id){
var info = $('info'+id);
if(!info){
http.makeRequest([id],'videos.updataList');
return;
}
if(info.style.display==='none'){
info.style.display = '';
}else{
info.style.display = 'none';
}
}
};
proxy.makeRequest(id,videos.updataList,videos);
js中介模式:
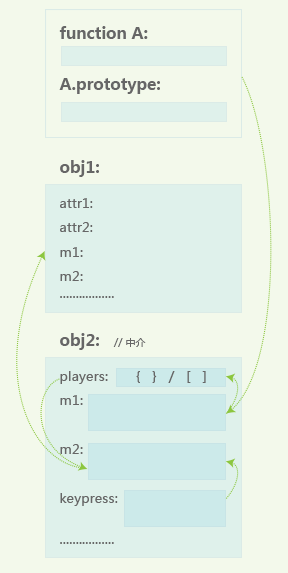
function Player(name){
this.points = 0;
this.name = name;
}
Player.prototype.play = function(){
this.points += 1;
mediator.played();
};
var scoreboard = {
element:document.getElementById('results'),
update:function(score){
var i,msg = '';
for(i in score){
if(score.hasOwnProperty(i)){
msg += '<p><strong>'+i+'</strong>:'+score[i]+'</p>';
}
}
this.element.innerHTML = msg;
}
};
var mediator = {
players:{},
setup:function(){
var players = this.players;
players.home = new Player('Home');
players.guest = new Player('Guest');
},
played:function(){
var players = this.players,
score = {
Home:players.home.points,
Guest:players.guest.points
};
scoreboard.update(score);
},
keypress:function(e){
e = e || window.event;
if(e.which === 49){
mediator.players.home.play();
return;
}
if(e.which === 48){
mediator.players.guest.play();
return;
}
}
};
mediator.setup();
window.onkeypress = mediator.keypress;
setTimeout(function(){
window.onkeypress = null;
alert('Game over!');
},30000);